This Codlet allows you to load state and city dropdown fields from country you select. It works as follow: 1. select country, states dropdown will be loaded from country. 2. select state, city dropdown will be loaded from state you select. Ex. selected country is 'INDIA' you get states dropdown depend on INDIA,
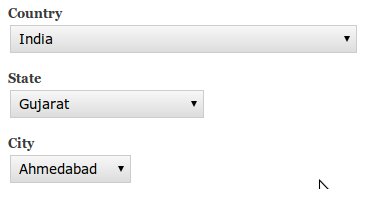
<?php
/**
* hook_form_alter
*/
function mymodule_form_alter(&$form, $form_state, $form_id) {
if($form_id == 'my_node_form') {
$country_default_value =$form_state['values']['field_cmn_city']['und']['0']['value'];
$state_default_value = $form_state['values']['field_cmn_state']['und']['0']['value'];
$city_default_value = $form_state['values']['field_cmn_city']['und']['0']['value'];
$country_options = _get_location('country');
$selected_country = $country_default_value;
$form['field_cmn_country']['und']['#options'] = $country_options;
$form['field_cmn_country']['und']['#default_value'] = $selected_country;
$form['field_cmn_country']['und']['#ajax'] = array(
'callback' => 'field_cmn_country_callback',
'wrapper' => 'field_cmn_state_replace',
);
$state_options = _get_location('state', $selected_country);// get state
$selected_state = $state_default_value;
$form['field_cmn_state']['und']['#prefix'] = '<div id="field_cmn_state_replace">';
$form['field_cmn_state']['und']['#suffix'] = '</div>';
$form['field_cmn_state']['und']['#options'] = $state_options;
$form['field_cmn_state']['und']['#default_value'] = $selected_state;
$form['field_cmn_state']['und']['#ajax'] = array(
'callback' => 'field_cmn_state_callback',
'wrapper' => 'field_cmn_city_replace',
);
$city_options = _get_location('city', $selected_state, $country_default_value);
$form['field_cmn_city']['und']['#prefix'] = '<div id="field_cmn_city_replace">';
$form['field_cmn_city']['und']['#suffix'] = '</div>';
$form['field_cmn_city']['und']['#options'] = $city_options;
$form['field_cmn_city']['und']['#default_value'] = $city_default_value;
}
}
function _get_location($type, $location_id = NULL, $country_id = NULL) {
switch ($type) {
case 'country':
return $country_options;
break;
case 'state':
return $state_options;
break;
case 'city':
return $city_options;
break;
}
// ajax country callback
function field_cmn_country_callback($form, $form_state) {
$form_field_state =$form['field_cmn_state'];
$form_field_city = $form['field_cmn_city'];
return array(
'#type' => 'ajax',
'#commands' => array(
ajax_command_replace("#field_cmn_state_replace", render($form_field_state)),
ajax_command_replace("#field_cmn_city_replace", render($form_field_city))
)
);
}
//ajax state callback
function field_cmn_state_callback($form, $form_state) {
return $form['field_cmn_city'];
}
?>