This CodeLet let you allow Remove tax line item from order.
Install module and make changes as per instruction on CodeLet.
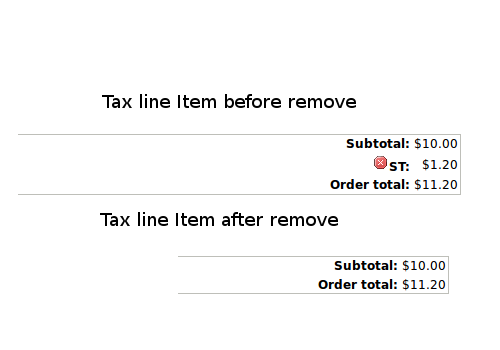
<?php//$Id$/** * @file * Custom Ubercart Tax remove module. * * Allows tax line item to be removed from orders. * @author saru1683 */function custom_uc_add_deleted_lineitem_order_id($line_item_id) { $sn__order_id = db_query("SELECT order_id FROM {uc_order_line_items} WHERE line_item_id = $line_item_id")->fetchField(); $data = array( 'order_id' => $sn__order_id, ); drupal_write_record('custom_uc_tax_line_item_deleted', $data); }function custom_check_deleted_item($order_id) { $sn__order_id = db_query("SELECT order_id FROM {custom_uc_tax_line_item_deleted} WHERE order_id = $order_id")->fetchField(); if(intval($sn__order_id) > 0) return TRUE; else return FALSE;}function custom_uc_tax_remove_form_alter($form, &$form_state, $form_id) { if($form_id == 'uc_order_edit_form') { //_pre($form['line_items']); }}?>
--------------- .module file end---------------
Setup Instruction
Step-1). Open a file "MODULE_PATH/ubercart/uc_order/uc_order.line_item.inc" on your favourite editor.
Find the function uc_order_delete_line_item($id, $order = FALSE) //Line No:91 and make change as bellowed example.
<?phpfunction uc_order_delete_line_item($id, $order = FALSE) { if ($order === FALSE) { if(module_exists('custom_uc_tax_remove'))// add this line for taxes remove custom_uc_add_deleted_lineitem_order_id($id);// add this line for taxes remove db_delete('uc_order_line_items') ->condition('line_item_id', $id) ->execute(); } else { db_delete('uc_order_line_items') ->condition('order_id', $id) ->execute(); } return TRUE;}?>
Step-2). Open a file "MODULE_PATH/ubercart/uc_order/uc_order.order_pane.inc" on your favourite editor.
Find the function uc_order_pane_line_items($op, $order, &$form = NULL, &$form_state = NULL) //Line no: 636 and make change as bellowed example.
<?phpfunction uc_order_pane_line_items($op, $order, &$form = NULL, &$form_state = NULL) { switch ($op) { ... ... case 'edit-form': ... ... foreach ($items as $item) { if (isset($item['add_list']) && $item['add_list'] === TRUE) { $options[$item['id']] = check_plain($item['title']); } if (isset($item['display_only']) && $item['display_only'] == TRUE) { $result = $item['callback']('display', $order); if (is_array($result)) { foreach ($result as $line) { if($line['id'] == 'tax' && module_exists('custom_uc_tax_remove') && custom_check_deleted_item($order->order_id)) {// add this line to remove taxes //do nothing } else {// add this line to remove taxes $line_items[] = array( 'line_item_id' => $line['id'], 'title' => $line['title'], 'amount' => $line['amount'], 'weight' => $item['weight'], ); }// add this line to remove taxes } } } } ... ...}?>
Step-3). Open a file "MODULE_PATH/ubercart/uc_taxes/uc_taxes.modules" on your favourite editor.
Find a function uc_taxes_uc_order($op, $order, $arg2) Line no:183 and make change as bellowed example.
<?phpfunction uc_taxes_uc_order($op, $order, $arg2) { switch ($op) { ... ... // Now add line items for any remaining new taxes. if (is_array($line_items)) { if(module_exists('custom_uc_tax_remove') && custom_check_deleted_item($order->order_id)) {// add this line to remove taxes } else { // add this line to remove taxes foreach ($line_items as $line) { $order->line_items[] = uc_order_line_item_add($order->order_id, 'tax', $line['title'], $line['amount'], $line['weight'], $line['data']); $changes[] = t('Added %amount for %title.', array('%amount' => uc_currency_format($line['amount']), '%title' => $line['title'])); } }// add this line to remove taxes } if (count($changes)) { uc_order_log_changes($order->order_id, $changes); usort($order->line_items, 'uc_weight_sort'); } break; }}?>
custom_uc_tax_remove.info
name = "Custom Ubercart"
description = "Customization on core Ubercart tax line item remove functionality from admin"
core = 7.x
package = "custom"
<?php//$Id$/** * @file * * hook = mg_permission * * @author JCS *//** * Implementation of hook_install * * @return unknown_type */function custom_uc_tax_remove_install() { drupal_install_schema('custom_uc_tax_line_item_deleted');}/** * Implementation of hook_uninstall * * @return unknown_type */function custom_uc_tax_remove_uninstall() { drupal_uninstall_schema('custom_uc_tax_line_item_deleted');}/** * Implementation of hook_schema * * * @return unknown_type */function custom_uc_tax_remove_schema() { $schema['custom_uc_tax_line_item_deleted'] = array( 'description' => t("Store file permissions"), 'fields' => array( 'custom_id' => array( 'type' => 'serial', 'length' => 11, 'not null' => TRUE, 'unsigned' => TRUE, ), 'order_id' => array( 'type' => 'int', 'length' => 11, 'not null' => TRUE, 'unsigned' => TRUE, ), ), 'primary key' => array('custom_id'), ); return $schema;}?>