This CodeLet will create achievements using Achievements API provided by Achievements module. The CodeLet describes how to use the API and build the custom Achievements badges for different activities on the site by the users. Based on these activities, a user will be awarded with an achievement badge. The CodeLet uses different Drupal API hooks in order to check whether the user has peformed an activity and is eligible to get the respective achievement badge.
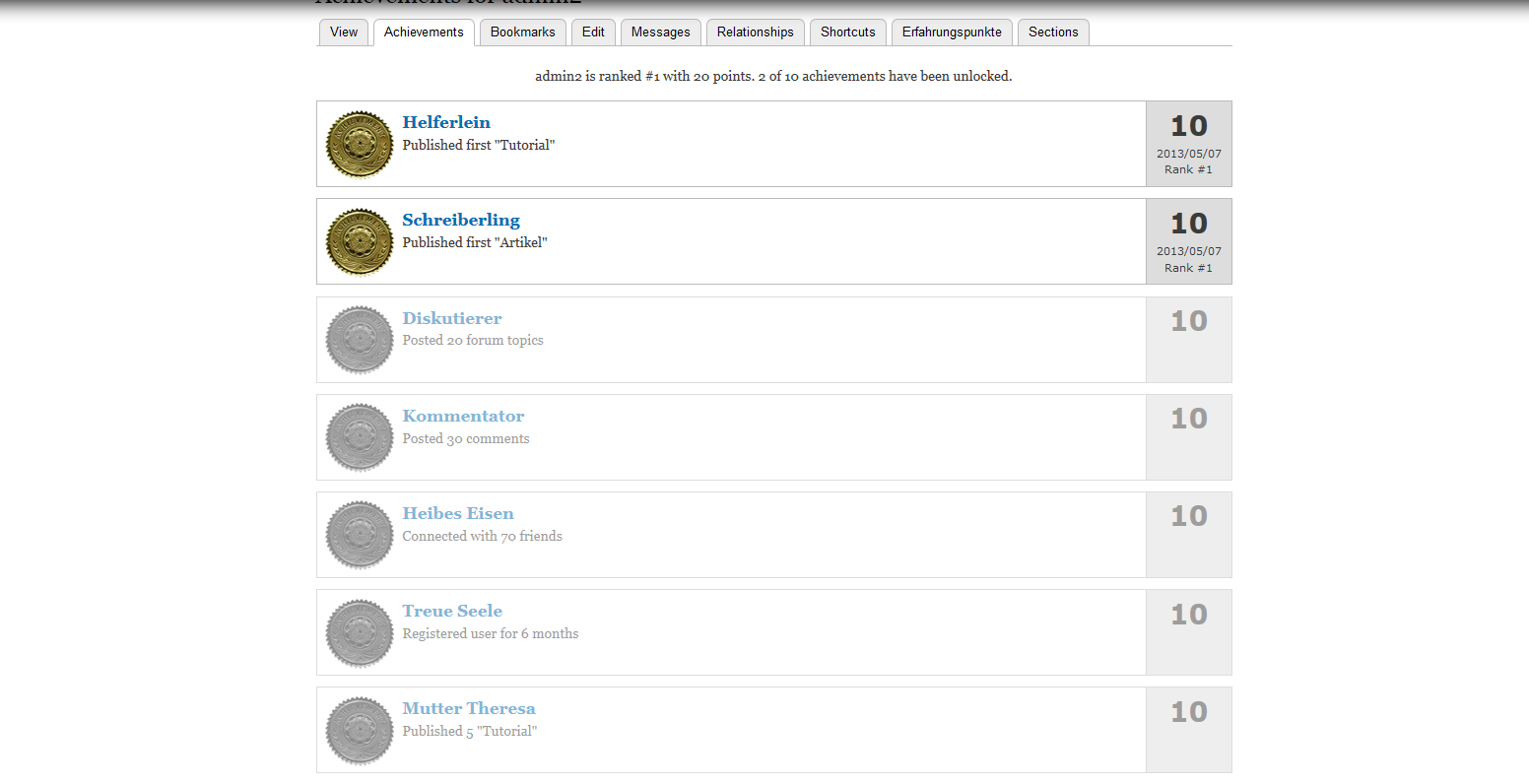
CodeLet
<?php/** * @file * * * @author DrupalD <DrupalD@twitter> *//** * Implemenation of hook_achievements_info * * @author DrupalD <DrupalD@twitter> */function geld_achievements_achievements_info() { $achievements = array( 'geld' => array( 'title' => t('Your achievements'), 'achievements' => array( 'diskutierer' => array( 'title' => t('Diskutierer'), 'description' => t('Posted 20 forum topics'), 'storage' => 'forum-node-count', 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'kommentator' => array( 'title' => t('Kommentator'), 'description' => t('Posted 30 comments'), 'storage' => 'comment-count', 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') .'/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') .'/images/achievement_locked-70.jpg', ), ), 'eisen' => array( 'title' => t('Heibes Eisen'), 'description' => t('Connected with 70 friends'), 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'seele' => array( 'title' => t('Treue Seele'), 'description' => t('Registered user for 6 months'), 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'helferlein' => array( 'title' => t('Helferlein'), 'description' => t('Published first "Tutorial"'), 'storage' => 'tutorial-node-count', 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'theresa' => array( 'title' => t('Mutter Theresa'), 'description' => t('Published 5 "Tutorial"'), 'storage' => 'tutorial-node-count', 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'schreiberling' => array( 'title' => t('Schreiberling'), 'description' => t('Published first "Artikel"'), 'storage' => 'artikel-node-count', 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'vielschreiber' => array( 'title' => t('Vielschreiber'), 'description' => t('Published 10 "Artikel"'), 'storage' => 'artikel-node-count', 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'verlinker' => array( 'title' => t('Verlinker'), 'description' => t('Linked to this site'), 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), 'kameramann' => array( 'title' => t('Kameramann'), 'description' => t('published first "Video-Inhalt"'), 'storage' => 'video-node-count', 'points' => 10, 'images' => array( 'unlocked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_unlocked-70.jpg', 'locked' => drupal_get_path('module', 'geld_achievements') . '/images/achievement_locked-70.jpg', ), ), ), ), ); return $achievements;}/** * Implementation of hook_comment_insert * * @param $comment * @author DrupalD <DrupalD@twitter> */function geld_achievements_comment_insert($comment) { $current_count = achievements_storage_get('comment-count', $comment->uid) + 1; achievements_storage_set('kommentator', $comment->uid); if ($current_count == 30) { achievements_unlocked('kommentator', $comment->uid); }}/** * Implementation of hook_node_insert * * @param $node * @author DrupalD <DrupalD@twitter> */function geld_achievements_node_insert($node) { switch ($node->type) { case 'forum': $current_count = achievements_storage_get('forum-node-count', $node->uid) + 1; achievements_storage_set('forum-node-count', $current_count, $node->uid); if ($current_count == 20) { achievements_unlocked('diskutierer', $node->uid); } break; case 'tutorial': $current_count = achievements_storage_get('tutorial-node-count', $node->uid) + 1; achievements_storage_set('tutorial-node-count', $current_count, $node->uid); if ($current_count == 1) { achievements_unlocked('helferlein', $node->uid); } if ($current_count == 5) { achievements_unlocked('theresa', $node->uid); } break; case 'article': $current_count = achievements_storage_get('artikel-node-count', $node->uid) + 1; achievements_storage_set('artikel-node-count', $current_count, $node->uid); if ($current_count == 1) { achievements_unlocked('schreiberling', $node->uid); } if ($current_count == 10) { achievements_unlocked('vielschreiber', $node->uid); } break; case 'video_inhalte': $current_count = achievements_storage_get('video-node-count', $node->uid) + 1; achievements_storage_set('video-node-count', $current_count, $node->uid); if ($current_count == 1) { achievements_unlocked('kameramann', $node->uid); } break; }}/** * Implementaiton of hook_user_login * * @param $edit * @param $account * @author DrupalD <DrupalD@twitter> */function geld_achievements_user_login(&$edit, $account) { $created = $account->created; $now = time('now'); $diff = abs($now - $created); $years = floor($diff / (365*60*60*24)); $months = floor(($diff - $years * 365*60*60*24) / (30*60*60*24)); if ($months >= 6) { achievements_unlocked('seele', $account->uid); } $requests = user_relationships_load($param = array("approved" => 1, "user" => $account->uid), $options = array(), $reset = FALSE); if(count($requests) >= 70) { achievements_unlocked('eisen', $account->uid); } }?>