This CodeLet recycles coupons created using Ubercart coupon module. The CodeLet chooses a coupon randomly from the available active coupons and send an email with coupon details to respective users. The coupon is remain active uintil defined period and then expires. This CodeLet, when cron runs, activates those coupons and those can be used again.
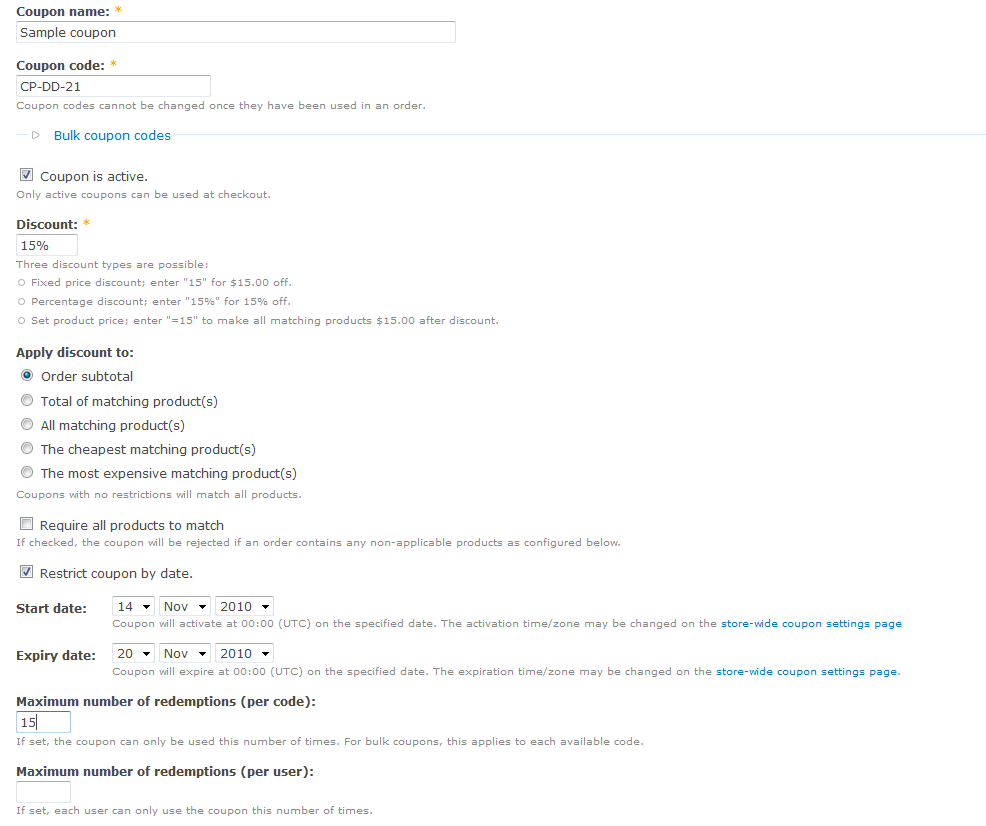
CodeLet
<?php//$Id$/** * @file * * Enhancements for exisiting features for CandyCulture. * * @author DrupalD <DrupalD@twitter> *//** * Implementation of hook_mail_alter * * @param unknown_type $message * @author DrupalD <DrupalD@twitter> */function candyculture_util_mail_alter(&$message) { switch ($message['id']) { case 'simplenews_node': case 'simplenews_test': $sn__nid = $message['params']['context']['node']->nid; $account = $message['params']['account']; if (!$sn__nid) { $sn__nid = db_result(db_query("SELECT nid FROM {simplenews_mail_spool} WHERE mail= '%s'", $account->mail)); } $am__inactive_coupon = _get_candyculture_util_inactive_coupon($sn__nid, $account); if ($am__inactive_coupon['cid']) { $am__tokens = array( '[coupon-code]' => $am__inactive_coupon['code'], '[coupon-name]' => $am__inactive_coupon['name'], '[coupon-value]' => $am__inactive_coupon['value'], ); $message['body']['body'] = t($message['body']['body'], $am__tokens); $ss__subject = t($message['subject'], $am__tokens); $message['subject'] = strip_tags($ss__subject); db_query("UPDATE {uc_coupons} SET status = 1, valid_from = %d, valid_until = %d WHERE cid = %d", strtotime('now'), strtotime('+1 week'), $am__inactive_coupon['cid']); } break; };}/** * _get_candyculture_util_inactive_coupon * * Choose and return an inactive coupon randomly * * @author DrupalD <DrupalD@twitter> */function _get_candyculture_util_inactive_coupon($sn__nid, $account) { $sn__nid = db_result(db_query("SELECT DISTINCT nid FROM {simplenews_mail_spool} WHERE mail= '%s' ORDER BY timestamp DESC", $account->mail)); $sn__uc_id = db_result(db_query("SELECT uc_id FROM {candy_coupon} WHERE nid = %d", $sn__nid)); if ($sn__uc_id) { $r__coupon = db_query("SELECT uc.cid, uc.code, uc.name, uc.value FROM {uc_coupons} uc LEFT JOIN {candy_coupon} cc ON uc.cid = cc.coupon_id WHERE sent = 0 AND nid = %d", $sn__nid); $am__coupon = db_fetch_array($r__coupon); } else { $r__result_cid = db_query('SELECT cid FROM {uc_coupons} WHERE status = 0'); while ($om__coupon_cid = db_fetch_object($r__result_cid)) { $am__cid[] = $om__coupon_cid->cid; } $sn__cid_index = count($am__cid) > 1 ? rand(0, count($am__cid) - 1) : 0; $sn__cid = $am__cid[$sn__cid_index]; $ss__coupon_code = _get_candyculture_util_coupon(); db_query('INSERT INTO {candy_coupon} VALUES(uc_id, %d, %d, 0)', $sn__cid, $sn__nid); db_query("UPDATE {uc_coupons} SET code = '%s' WHERE cid = %d", $ss__coupon_code, $sn__cid); $r__result = db_query("SELECT cid, name, code, value FROM {uc_coupons} WHERE status = 0 AND cid = %d", $sn__cid); $am__coupon = db_fetch_array($r__result); } return $am__coupon;}/** * _get_candyculture_util_coupon * * Return a reandomly generated code * * @author DrupalD <DrupalD@twitter> */function _get_candyculture_util_coupon() { $sn__index = rand(65, 90); $ss__char = chr($sn__index); foreach (range('A', $ss__char) as $ss__letter) { if (is_scalar($ss__letter)) { $as__code[] = $ss__letter; } } $an__code = range(65, $sn__index); shuffle($as__code); shuffle($an__code); $ss__code = substr(implode('', $as__code), rand(1, count($as__code) - 3), 3); $sn__code = substr(implode('', $an__code), rand(1, count($an__code) - 5), 5); $ss__coupon_code = $ss__code .''. $sn__code; return $ss__coupon_code; }/** * Implementation of hook_cron * * @author DrupalD <DrupalD@twitter> */function candyculture_util_cron() { db_query("UPDATE {uc_coupons} SET status = 0 WHERE valid_until <= %d AND status = 1", strtotime('now')); db_query("UPDATE {uc_coupons} SET valid_from = 0, valid_until = 0 WHERE status = 0"); db_query('TRUNCATE TABLE {candy_coupon}');}?>
Install file details
<?php//$Id$/** * @file * * Schema definiation for Candyculture utility * * @author DrupalD <DrupalD@twitter> *//** * Implementation of hook_install * * @author DrupalD <DrupalD@twitter> */function candyculture_util_install() { global $b__lock; $b__lock = FALSE; drupal_install_schema('candyculture_util');}/** * Implemeantion of hook_uninstall_schema * * @author DrupalD <DrupalD@twitter> */function candyculture_util_uninstall() { drupal_uninstall_schema('candyculture_util');}/** * Implementation of hook_schema * * @author DrupalD <DrupalD@twitter> */function candyculture_util_schema() { $schema = array(); $schema['candy_coupon'] = array( 'fields' => array( 'uc_id' => array( 'type' => 'serial', 'length' => 11, 'not null' => TRUE, ), 'coupon_id' => array( 'type' => 'int', 'length' => 11, 'not null' => TRUE, ), 'nid' => array( 'type' => 'int', 'length' => 11, 'not null' => TRUE, ), 'sent' => array( 'type' => 'int', 'length' => 1, 'not null' => TRUE, ), ), 'primary key' => array('uc_id'), ); return $schema;}?>