This CodeLet will generate the number of Drupal sub sites you specify. It requires path to mysql executable on your server. The sub sites will be pre-populated with an SQL file.
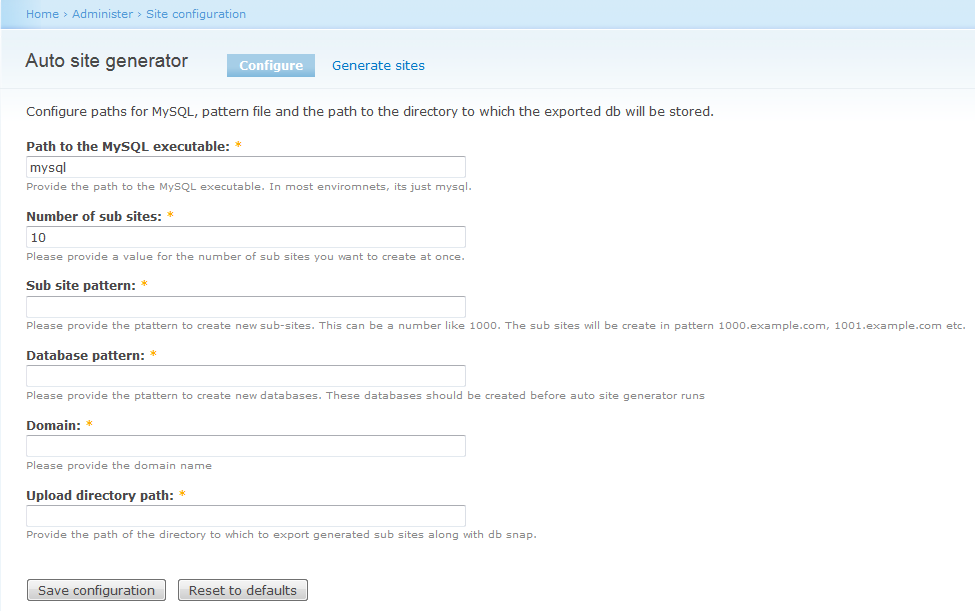
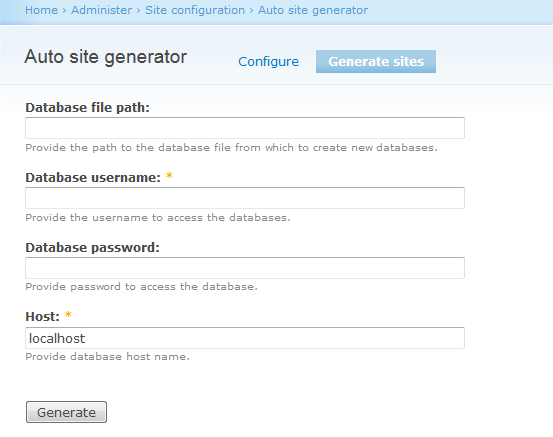
<?php
//$Id$
/**
* @file
*
* Automtically generate sub site with prepopulated identical databases
*
* @author DrupalD
*/
/**
* Imeplementation of hook_help
*
* @param string $path
* @author DrupalD
*/
function auto_site_help($path) {
switch ($path) {
case 'admin/settings/auto-site':
$output = "
". t("Configure paths for MySQL, pattern file and the path to the directory to which the exported db will be stored.") ."
";
return $output;
}
}
/**
* Implementation of hook_menu
*
* @author DrupalD
*/
function auto_site_menu() {
$items = array();
$items['admin/settings/auto-site'] = array(
'title' => 'Auto site generator',
'title callback' => 't',
'page callback' => 'drupal_get_form',
'page arguments' => array('auto_site_admin_form'),
'access arguments' => array('Administer auto site generator'),
);
$items['admin/settings/auto-site/configure'] = array(
'type' => MENU_DEFAULT_LOCAL_TASK,
'title' => 'Configure',
'title callback' => 't',
'page callback' => 'drupal_get_form',
'page arguments' => array('auto_site_admin_form'),
'access arguments' => array('Administer auto site generator'),
'weight' => -10,
);
$items['admin/settings/auto-site/generate'] = array(
'type' => MENU_LOCAL_TASK,
'title' => 'Generate sites',
'title callback' => 't',
'page callback' => 'drupal_get_form',
'page arguments' => array('auto_site_genrate_form'),
'access arguments' => array('Administer auto site generator'),
'weight' => -9
);
return $items;
}
/**
* Implementation of hook_perm
*
* @author DrupalD
*/
function auto_site_perm() {
return array(t("Administer auto site generator"));
}
/**
* auto_site_admin_form
*
* Return configuration form for auto site
*
* @author DrupalD
*/
function auto_site_admin_form() {
$form = array();
$form['auto_mysql_path'] = array(
'#type' => 'textfield',
'#title' => t("Path to the MySQL executable"),
'#default_value' => variable_get('auto_mysql_path', 'mysql'),
'#description' => t("Provide the path to the MySQL executable.
In most enviromnets, its just mysql."),
'#required' => TRUE,
'#weight' => -10,
);
$form['auto_site_pattern'] = array(
'#type' => 'textfield',
'#title' => t("Sub site pattern"),
'#default_value' => variable_get("auto_site_pattern", ''),
'#description' => t("Please provide the ptattern to create new sub-sites.
This can be a number like 1000. The sub sites will be create in pattern 1000.example.com, 1001.example.com etc. "),
'#required' => TRUE,
'#weight' => -9,
);
$form['auto_site_count'] = array(
'#type' => 'textfield',
'#title' => t("Number of sub sites"),
'#default_value' => variable_get("auto_site_count", 10),
'#description' => t("Please provide a value for the number of sub sites you want to create at once."),
'#required' => TRUE,
'#weight' => -9,
);
$form['auto_db_pattern'] = array(
'#type' => 'textfield',
'#title' => t("Database pattern"),
'#default_value' => variable_get("auto_db_pattern", ''),
'#description' => t("Please provide the ptattern to create new databases. These databases should be created before auto site generator runs"),
'#required' => TRUE,
'#weight' => -8,
);
$form['auto_domain'] = array(
'#type' => 'textfield',
'#title' => t("Domain"),
'#default_value' => variable_get("auto_domain", ''),
'#description' => t("Please provide the domain name"),
'#required' => TRUE,
'#weight' => -7,
);
$form['auto_upload_path'] = array(
'#type' => 'textfield',
'#title' => t("Upload directory path"),
'#default_value' => variable_get("auto_upload_path", ''),
'#description' => t("Provide the path of the directory to which to export generated sub sites along with db snap."),
'#required' => TRUE,
'#weight' => -6,
);
return system_settings_form($form);
}
function auto_site_genrate_form() {
$form = array();
$form['auto_mysql_db'] = array(
'#type' => 'textfield',
'#title' => t("Database file path"),
'#description' => t("Provide the path to the database file from which to create new databases."),
'#weight' => -10,
);
$form['auto_mysql_username'] = array(
'#type' => 'textfield',
'#title' => t("Database username"),
'#description' => t("Provide the username to access the databases."),
'#required' => TRUE,
'#weight' => -9,
);
$form['auto_mysql_password'] = array(
'#type' => 'password',
'#title' => t("Database password"),
'#description' => t("Provide password to access the database."),
'#weight' => -8,
);
$form['auto_mysql_host'] = array(
'#type' => 'textfield',
'#title' => t("Host"),
'#description' => t("Provide database host name."),
'#default_value' => 'localhost',
'#required' => TRUE,
'#weight' => -7,
);
$form['generate'] = array(
'#type' => 'submit',
'#value' => t("Generate"),
);
$form['#submit'] = array('auto_site_generate');
return $form;
}
/**
* auto_site_generate
*
* Form submit handler
*
* @param $form
* @param $form_values
* @author DrupalD
*/
function auto_site_generate($form, $form_values) {
require_once ("includes/install.inc");
$ss__site_pattern = variable_get('auto_site_pattern', '');
$ss__site_count = variable_get('auto_site_count', 10);
$ss__domain = variable_get('auto_domain', '');
if ($ss__site_pattern) {
$ss__db_pattern = variable_get('auto_db_pattern', '');
$ss__mysql_path = variable_get('auto_mysql_path', '');
$ss__upload_path = variable_get('auto_upload_path', '');
$ss__db_path = $form_values['values']['auto_mysql_db'];
$ss__db_user = $form_values['values']['auto_mysql_username'];
$ss__db_password = $form_values['values']['auto_mysql_password'];
$ss__db_host = $form_values['values']['auto_mysql_host'];
$ss__source = "sites/default/default.settings.php";
$ss__db = $ss__db_pattern .".". $ss__domain;
if ($ss__db_password) {
$ss__db_string = '$db_url ='. "\'mysqli://$ss__db_user:$ss__db_password@$ss__db_host/$ss__db\';";
}
else {
$ss__db_string = '$db_url = '. "'mysqli://$ss__db_user@$ss__db_host/$ss__db';";
}
for ($i = $ss__site_pattern; $i < ($ss__site_pattern + $ss__site_count); $i++) {
$ss__path = $ss__upload_path ."/". $i . ".". $ss__domain;
$ss__file_path = $ss__path ."/files";
file_check_directory($ss__path, 1);
$h__write_file = fopen($ss__path ."/settings.php", "w+");
$h__read_file = fopen($ss__source, "r");
while (!feof($h__read_file)) {
$output = fread($h__read_file, 4096);
$output = preg_replace('/\$db_url.*/', $ss__db_string, $output);
fwrite($h__write_file, $output, 4096);
}
fclose($h__read_file);
fclose($h__write_file);
file_check_directory($ss__file_path, 1);
if ($ss__db_password) {
exec("$ss__mysql_path --default-character-set=utf8 -h $ss__db_host -u $ss__db_user --password= $ss__db_password ". $ss__site_pattern. "_dd" ." < $ss__db_path 2<&1", $output, $return);
}
else {
exec("$ss__mysql_path --default-character-set=utf8 -h $ss__db_host -u $ss__db_user ". $ss__site_pattern. "_dd" ." < $ss__db_path 2<&1" , $output, $return);
}
}
}
}
?>