This CodeLet enables a Drupal site to moderate the comments automatically. Once a user's comment has been white lited, all his/her furture comments will be automatically moderated without admin to review them.
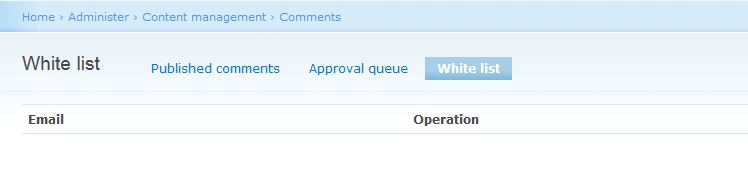
Module file
<?php
//$Id$
/**
* @file
*
* Allow white listed anonymous users to post comments without approval
*
* @author DrupalD
*/
/**
* Implementation of hook_menu
*
* @author DrupalD
*/
function whitelist_comment_menu() {
$item = array();
$item['admin/content/comment/whitelist'] = array(
'type' => MENU_LOCAL_TASK,
'title' => t('White list'),
'page callback' => 'whitelist_comment_list',
'access arguments' => array('administer comments'),
'weight' => 0,
);
$item['whitelist/%/delete'] = array(
'type' => MENU_CALLBACK,
'page callback' => 'drupal_get_form',
'page arguments' => array('whitelist_comment_confirm_form', 1),
'access arguments' => array('administer comments'),
);
return $item;
}
/**
* Implementation of hook_form_FORM_ID_alter
*
* @param unknown_type $form
* @param unknown_type $form_values
* @author Bhvain H. Joshi
*/
function whitelist_comment_form_comment_form_alter(&$form, $form_values) {
//unset the name field value on comment form
$form['name']['#default_value'] = '';
}
/**
* Implementation of hook_comment
*
* @param $a1
* @param $op
* @author DrupalD
*/
function whitelist_comment_comment(&$a1, $op) {
switch ($op) {
case 'publish':
if ($a1->uid == 0) {
$ss__email = db_result(db_query("SELECT mail FROM {whitelist_comment} WHERE mail = '%s'", $a1->mail));
if (empty($ss__email)) {
db_query("INSERT INTO {whitelist_comment} VALUES (whitelist_id, '%s')", $a1->mail);
}
}
break;
case 'insert':
global $user;
if ($user->uid == 0) {
$ss__email = db_result(db_query("SELECT mail FROM {whitelist_comment} WHERE mail = '%s'", $a1['mail']));
if (!empty($ss__email)) {
db_query("UPDATE {comments} SET status = 0 WHERE mail = '%s'", $ss__email);
}
}
break;
}
}
/**
* whitelist_comment_list
*
* List all the whitelisted emails
*
* @author DrupalD
*/
function whitelist_comment_list() {
drupal_set_title(t('White list'));
$header = array(t('Email'), t('Operation'));
$r__result = db_query("SELECT * FROM {whitelist_comment}");
while ($om__result = db_fetch_object($r__result)) {
$row[] = array(
$om__result->mail,
l(t('Remove'), 'whitelist/'. $om__result->whitelist_id .'/delete'),
);
}
return theme('table', $header, $row);
}
/**
* whitelist_comment_confirm_form
*
* Display a confirmation form to remove the whitelisted email
*
* @param $form_values
* @param $sn__whitelist_id
* @author Bhavin H. Joshi
*/
function whitelist_comment_confirm_form($form_values = NULL, $sn__whitelist_id) {
$form['#submit'] = array('whitelist_comment_confirm_remove');
$form['whitelist_id'] = array(
'#type' => 'hidden',
'#default_value' => $sn__whitelist_id,
);
$ss__email = db_result(db_query("SELECT mail FROM {whitelist_comment} WHERE whitelist_id = %d", $sn__whitelist_id));
$question = t('Are you sure you want to remove white listed email %email?', array('%email' => $ss__email));
$path = 'admin/content/comment/whitelist';
$yes = t('Remove');
return confirm_form($form, $question, $path, NULL, $yes);
}
/**
* whitelist_comment_confirm_remove
*
* Remove the email after it has been confirmed
*
* @param unknown_type $form
* @param unknown_type $form_values
* @author DrupalD
*/
function whitelist_comment_confirm_remove($form, $form_values) {
db_query("DELETE FROM {whitelist_comment} WHERE whitelist_id = %d", $form_values['values']['whitelist_id']);
drupal_set_message(t('White listed email has been removed successfully'));
drupal_goto('admin/content/comment/whitelist');
}
?>
Install file
<?php
//$Id$
/**
* @file
*
* Create schema for whitelist comment
*
* @author DrupalD
*/
/**
* Implementation of hook_install
*
* @author DrupalD
*/
function whitelist_comment_install() {
drupal_install_schema('whitelist_comment');
}
/**
* Implementation of hook_uninstall
*
* @author DrupalD
*/
function whitelist_comment_uninstall() {
drupal_uninstall_schema('whitelist_comment');
}
/**
* Implementation of hook_schema
*
* @author DrupalD
*/
function whitelist_comment_schema() {
$schema = array();
$schema['whitelist_comment'] = array(
'description' => t('A list of moderated emails'),
'fields' => array(
'whitelist_id' => array(
'type' => 'serial',
'length' => 11,
'not null' => TRUE,
),
'mail' => array(
'type' => 'text',
'not null' => TRUE,
),
),
'primary key' => array('whitelist_id'),
);
return $schema;
}
?>