This CodeLet reads details from the uploaded CSV file and import the data as nodes in respective fields. The fields can be CCK fields. Using the CodeLet, the CSV files are uploaded and saved to a location and nodes are created from it.
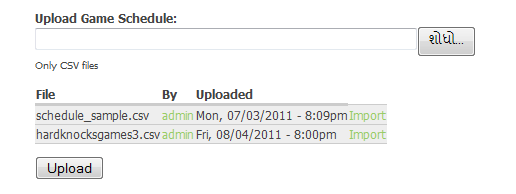
<?php
//$Id$
/**
* @file
*
* Import game schedules
*
* @author DrupalD
*/
/**
* Implementation of hook_menu
*
* @author DrupalD
*/
function game_schedule_menu() {
$item = array();
$item['import-schedule'] = array(
'title' => 'Upload Game Schedule',
'title callback' => 't',
'page callback' => 'drupal_get_form',
'page arguments' => array('game_schedule_upload'),
'access arguments' => array('Import Game schedule'),
);
$item['schedule/%'] = array(
'type' => MENU_CALLBACK,
'page callback' => '_game_schedule_process',
'page arguments' => array(1),
'access arguments' => array('Import Game schedule'),
);
return $item;
}
/**
* Implementation of hook_param
*
* @author DrupalD
*/
function game_schedule_upload_param() {
return array('Import Game schedule');
}
/**
* game_schedule_upload
*
* Upload game schedule
*
* @author DrupalD
*/
function game_schedule_upload() {
$form = array();
$form['#attributes'] = array('enctype' => "multipart/form-data");
$form['import_schedule'] = array(
'#type' => 'file',
'#title' => t('Upload Game Schedule'),
'#description' => t('Only CSV files'),
);
$form['history'] = array(
'#type' => 'item',
'#value' => _game_schedule_history(),
);
$form['submit'] = array(
'#type' => 'submit',
'#value' => t('Upload'),
);
$form['#submit'] = array('game_schedule_upload_save');
return $form;
}
/**
* game_schedule_upload_save
*
* Import records in the database
*
* @param $form
* @param $form_values
* @author DrupalD
*/
function game_schedule_upload_save($form, $form_values) {
global $user;
$ss__destination = file_directory_path() ."/game_schedule";
if (file_check_directory($ss__destination, 1)) {
if ($om__file = file_save_upload('import_schedule', array(), $ss__destination, FILE_EXISTS_RENAME)) {
$ss__query = "INSERT INTO {game_schedule_history} VALUES(sid, %d, '%s', %d)";
db_query($ss__query, $user->uid, $om__file->filepath, time());
file_set_status($om__file, 1);
drupal_set_message(t('!file uploaded successfully', array('!file' => $om__file->filename)));
}
else {
drupal_set_message(t('Problem while uploading the file'), 'error');
}
}
else {
drupal_set_message(t('!destination is not writable', array('!destination' => $ss__destination)), 'error');
}
}
/**
* _game_schedule_history
*
* Return previously uploaded game schedule
*
* @author DrupalD
*/
function _game_schedule_history() {
$header = array(t('File'), t('By'), t('Uploaded'));
$r__result = db_query("SELECT fid, files.filename, gsh.uid, uploaded
FROM {game_schedule_history} gsh LEFT JOIN {files} files ON gsh.filepath = files.filepath");
while ($om__result = db_fetch_object($r__result)) {
$om__user = user_load($om__result->uid);
$row[] = array(
$om__result->filename,
l($om__user->name, "user/". $om__user->uid),
format_date($om__result->uploaded, 'medium'),
l(t('Import') , "schedule/". $om__result->fid),
);
}
return theme('table', $header, $row);
}
/**
* _game_schedule_process
*
* Import game schedules
*
* @param integer $sn__fid
* @author DrupalD
*
*/
function _game_schedule_process($sn__fid) {
global $user;
$ss__filepath = db_result(db_query("SELECT filepath FROM {files} WHERE fid = %d", $sn__fid));
$h__file = fopen($ss__filepath, "r+");
$ss__header = stream_get_line($h__file, 1024, "\r\n");
$i = 0;
while (!feof($h__file)) {
$i++;
$row = stream_get_line($h__file, 1024, "\r\n");
$row = preg_replace('/"/', "", $row);
list($ss__date, $ss__time, $ss__visitor, $ss__home, $ss__location, $sn__field, $ss__division, $ss__program) = explode(",", $row);
db_query("INSERT INTO {game_schedule} VALUES(sid, %d, %d, '%s', '%s', '%s', %d, '%s', '%s')",
strtotime($ss__date), strtotime($ss__time), $ss__visitor, $ss__home, $ss__location, $sn__field, $ss__division, $ss__program);
unset($row);
}
fclose($h__file);
if (db_affected_rows()) {
drupal_set_message(t('!row schedules imported successfully', array('!row' => $i)));
}
else {
drupal_set_message(t('Error while importing the schedules'), 'error');
}
$r__result = db_query("SELECT * FROM {game_schedule}");
while($om__result = db_fetch_object($r__result)) {
//Create node
$node = new StdClass();
$node->type = 'game';
$node->uid = 1;
$node->title = t('@date', array('@date' => format_date($om__result->date, 'custom', 'D, d/m/Y')));
//Date
$ss__date = format_date($om__result->date, 'custom', 'Y-m-d\TH:i:s');
$node->field_date = array(
0 => array(
'value' => $ss__date,
),
);
//Location
$node->field_location = array(
0 => array(
'value' => $om__result->location,
),
);
//Home team
$node->field_home = array(
0 => array(
'value' => $om__result->home,
),
);
//Visitor
$node->field_away = array(
0 => array(
'value' => $om__result->visitor,
),
);
//Division
$node->field_divsion = array(
0 => array(
'value' => $om__result->divisoin,
),
);
//Program
$node->field_program = array(
0 => array(
'value' => $om__result->program,
),
);
//Time
$node->field_time = array(
0 => array(
'value' => $om__result->time,
),
);
//Field
$node->field_number = array(
0 => array(
'value' => $om__result->field_nuber,
),
);
node_submit($node);
node_save($node);
drupal_set_message(db_error(), 'error');
drupal_set_message(t('@node has been created', array('@node' => $node->title)));
}
unset($node, $om__result, $r__result);
db_query("DELETE FROM {game_schedule}");
drupal_goto('import-schedule');
}
?>