This CodeLet pulls results for search string fron instagram by using Instagram API. The result is displayed in tile. Befor you use the code, you have to register your app at instagram and obtain a client id which should be used in application.js file used with current code.
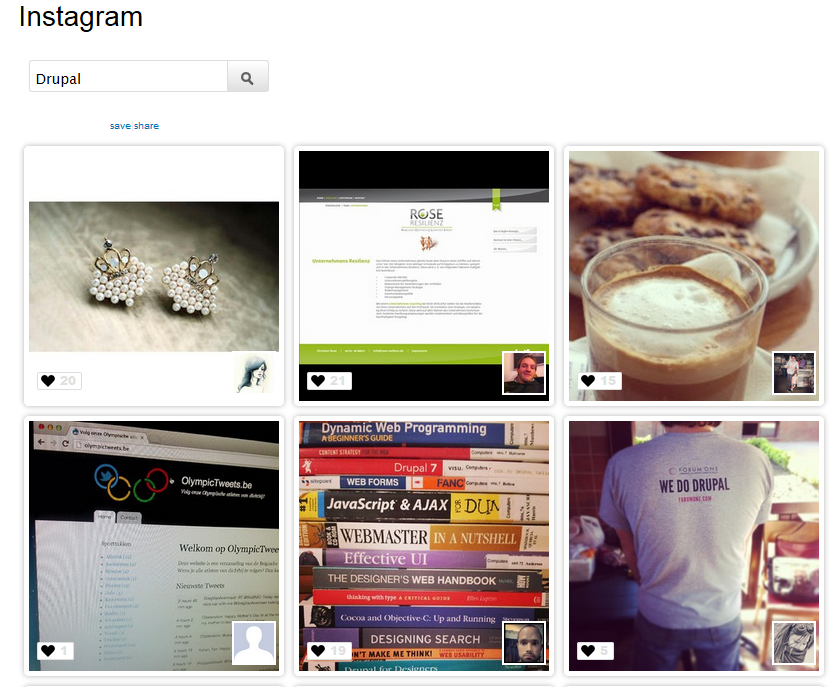
CodeLet
<?php /** * implements hook_menu() * * @author DrupalD <http://twitter.com/DrupalD> */function instasearch_menu(){ $items = array(); $items['instagram'] = array( 'title' => 'Instagram', 'page callback' => 'instagram_info', // after visit drupal6/person, instagram_info() function is called 'access callback' => true, // must return true, otherwise it will not visible as menu item 'type' => MENU_NORMAL_ITEM, // drupal's default menu type 'weight' => '10', // we want to display person link below in our nav menu ); $items['search_save'] = array( 'type' => MENU_CALLBACK, 'page callback' => '_instagram_save', 'access callback' => TRUE, ); $items['search_share'] = array( 'type' => MENU_CALLBACK, 'page callback' => '_instagram_share', 'access callback' => TRUE, ); $items['user/%/instagram'] = array( 'type' => MENU_LOCAL_TASK, 'title' => 'instagram', 'page callback' => '_instagram_user_search', 'access callback' => TRUE, ); $items['mashup/%'] = array( 'type' => MENU_CALLBACK, 'page callback' => '_insta_stats', 'page arguments' => array(1), 'access callback' => TRUE, ); return $items; // finally, do not forget to return $items array}/** * callback function for person * * @author DrupalD <http://twitter.com/DrupalD> */function instagram_info(){ $tag=''; $output = " <script type='text/javascript' src='https://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js'></script> <script src='sites/all/modules/instasearch/javascripts/application.js' type='text/javascript'></script> <script src='sites/all/modules/instasearch/javascripts/save-share.js' type='text/javascript'></script> <link rel='stylesheet' href='sites/all/modules/instasearch/stylesheets/application.css' type='text/css' media='screen'> <form id='search'> <button class='button' type='submit' id='search-button' dir='ltr' tabindex='2'> <span class='button-content'>Search</span> </button> <div class='search-wrap'> <div id='alert_message' style='height:auto;float:left;color:#000 !important;width:auto;'></div> <input class='search-tag' type='text' tabindex='1' value='".$tag."' /> <div class='fb-send' data-href='http://drupaldeveloper.in' data-font='lucida grande' data-colorscheme='light'></div> <span style='margin: 0 80px auto; font-size: 10px;float:left;'>". l(t('save') ,'', array('attributes' => array('id' => 'save_search'), 'fragment' => 'save')) ."|". l(t('share'), '', array('attributes' => array('id' => 'share_search'), 'fragment' => 'share')) ."</span> </div> </form> <div id='photos-wrap'> </div> <div class='paginate'> <a class='button' style='display:none;' data-max-tag-id='' href='#'>View More...</a></div> "; if (!empty($_GET['tag'])) { $tag = $_GET['tag']; $output .= "<script type='text/javascript'> $('input.search-tag').val('". $tag ."'); $('form#search-button').trigger('click'); </script>"; } return $output;}/** * Implements hook_block_info(). */function instasearch_block_info() { $blocks = array(); //block array$blocks['custom_bk'] = array( 'info' => t('instagram'), 'cache' => DRUPAL_NO_CACHE, ); return $blocks; } /** * _instagram_save * * @author DrupalD <http://twitter.com/DrupalD> */function _instagram_save() { global $user; $tag = $_GET['tag']; if ($user->uid > 0) { $sn__search_id = db_insert('insta_search') ->fields(array( 'search_tag' => $tag, 'uid' => $user->uid, 'search_time' => time('now'), )) ->execute(); print t('Your @tag search has been saved', array('@tag' => $tag)); } else { print t('Please login to save your search'); }}/** * _insta_stats * * @author DrupalD <http://twitter.com/DrupalD> */function _insta_stats($ss__string) { list($ss__tag, $ss__user)= explode('-', $ss__string); $result = db_select('insta_search', 'ins'); $result->leftjoin('users', 'u', 'ins.uid = u.uid'); $result->fields('ins', array('id')) ->condition('u.name', $ss__user, '='); $om__result = $result->execute(); $record = $om__result->fetchAssoc(); db_insert('insta_search_stats') ->fields(array( 'insta_search_id' => $record['id'], )) ->execute(); drupal_goto('instagram', array('query' => array('tag' => $ss__tag)));}/** * _instagram_user_search * * @author DrupalD <http://twitter.com/DrupalD> */function _instagram_user_search() { global $user; if ($user->uid == 0 && arg(1) != '') { $sn__uid = arg(1); $user = user_load($sn__uid); } else { $sn__uid = $user->uid; } $am__header = array(t('Search tag'), t('Search time'), t('Share string')); $result = db_select('insta_search', 'ins') ->fields('ins', array('search_tag', 'search_time')) ->condition('uid', $user->uid, '=') ->orderBy('search_time', 'DESC') ->execute(); while ($record = $result->fetchAssoc()) { $ss__share_url = "http://drupaldeveloper.in/mashup/". $record['search_tag'] .'-'. $user->name; $row[] = array( $record['search_tag'], format_date($record['search_time'], 'short'), "<div class='fb-send' data-href='". $ss__share_url ."' data-font='lucida grande' data-colorscheme='light'></div>" .' '. $ss__share_url, ); } if (empty($row)) { return t('You have not preformed any search yet!'); } else { return theme('table', array('header' => $am__header, 'rows' => $row)); } }/** * _instagram_share * * @author DrupalD <http://twitter.com/DrupalD> */function _instagram_share() { global $user; $tag = $_GET['tag']; print t('Your share string: !share', array('!share' => 'http://drupaldeveloper.in/mashup/'. $tag .'-'. $user->name)); exit;}?>
appilcation.js file:
// Instantiate an empty object.
var Instagram = {};
// Small object for holding important configuration data.
Instagram.Config = {
clientID: 'Your instagram app client id',
apiHost: 'https://api.instagram.com'
};
// Quick and dirty templating solution.
Instagram.Template = {};
Instagram.Template.Views = {
"photo": "<div class='photo'>" +
"<a href='{url}' target='_blank'><img class='main' src='{photo}' width='250' height='250' style='display:none;' onload='Instagram.App.showPhoto(this);' /></a>" +
"<img class='avatar' width='40' height='40' src='{avatar}' style='' onload='' />" +
"<span class='heart'><strong>{count}</strong></span>" +
"</div>"
};
Instagram.Template.generate = function(template, data){
var re, resource;
template = Instagram.Template.Views[template];
for(var attribute in data){
re = new RegExp("{" + attribute + "}","g");
template = template.replace(re, data[attribute]);
}
return template;
};
// ************************
// ** Main Application Code
// ************************
(function(){
function init(){
bindEventHandlers();
}
function toTemplate(photo){
photo = {
count: photo.likes.count,
avatar: photo.user.profile_picture,
photo: photo.images.low_resolution.url,
url: photo.link
};
return Instagram.Template.generate('photo', photo);
}
function toScreen(photos){
var photos_html = '';
$('.paginate a').attr('data-max-tag-id', photos.pagination.next_max_id)
.fadeIn();
$.each(photos.data, function(index, photo){
photos_html += toTemplate(photo);
});
$('div#photos-wrap').append(photos_html);
}
function generateResource(tag){
var config = Instagram.Config, url;
if(typeof tag === 'undefined'){
throw new Error("Resource requires a tag. Try searching for cats!");
} else {
// Make sure tag is a string, trim any trailing/leading whitespace and take only the first
// word, if there are multiple.
tag = String(tag).trim().split(" ")[0];
}
url = config.apiHost + "/v1/tags/" + tag + "/media/recent?callback=?&client_id=" + config.clientID;
return function(max_id){
var next_page;
if(typeof max_id === 'string' && max_id.trim() !== '') {
next_page = url + "&max_id=" + max_id;
}
return next_page || url;
};
}
function paginate(max_id){
$.getJSON(generateUrl(tag), toScreen);
}
function search(tag){
resource = generateResource(tag);
$('.paginate a').hide();
$('#photos-wrap *').remove();
fetchPhotos();
}
function fetchPhotos(max_id){
$.getJSON(resource(max_id), toScreen);
}
function bindEventHandlers(){
$('body').on('click', '.paginate a.button', function(){
var tagID = $(this).attr('data-max-tag-id');
fetchPhotos(tagID);
return false;
});
// Bind an event handler to the `click` event on the form's button
$('#search-button').click(function(){
// Extract the value of the search input text field.
var tag = $('input.search-tag').val();
// Invoke `search`, passing `tag`.
search(tag);
// Stop event propagation.
return false;
});
}
function showPhoto(p){
$(p).fadeIn();
}
Instagram.App = {
search: search,
showPhoto: showPhoto,
init: init
};
})();
$(function(){
Instagram.App.init();
// Start with a search on cats; we all love cats.
});
save-share.js file:
$(document).ready(function() {
$('#save_search').click(function() {
tag = $('input.search-tag').val();
$('#alert_message').empty();
$.ajax({
type: 'GET',
url: 'search_save',
data: 'tag=' + tag,
async: false,
success: function(msg) {
$('#alert_message').text(msg).show('slow');
}
}).responseText;
});
$('#share_search').click(function() {
tag = $('input.search-tag').val();
$('#alert_message').empty();
$.ajax({
type: 'GET',
url: 'search_share',
data: 'tag=' + tag,
async: false,
success: function(msg) {
$('#alert_message').text(msg).show('slow');
}
}).responseText;
});
});
You can tweak the code in the way you want!